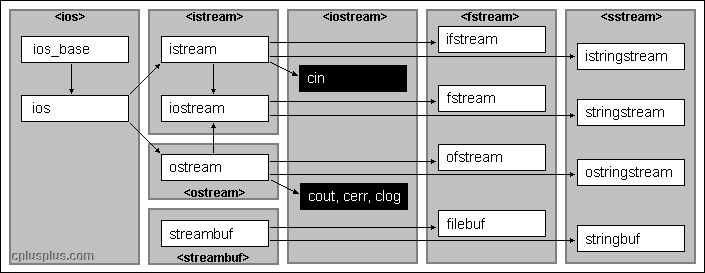 |
iostream hierarchy of classes (char instantiation):
ios_base,
ios,
istream,
ostream,
iostream,
streambuf,
ifstream,
fstream,
ofstream,
filebuf,
istringstream,
stringstream,
ostringstream,
stringbuf
|
The iostream library is an object-oriented library that provides input and
output functionality using streams.
A stream is an abstraction that represents a device in which input and ouput
operations are performed.
A stream can basically be represented as an indefinite source or destination
of characters - the number of character to be in/output is not needed to be known
in advance - We can simply write characters
to the stream or get them until we find it convenient or until the source of characters
exhausts.
The streams are generally associated to a physical source or destination of characters,
like a disk file, the keyboard or a text console, so the characters we get or
write to/from our abstraction called stream are physically input/output to the
physical device. For example, file streams are C++ objects to manipulate
and interact with files; Once a file stream is used to open a file, any input or
output operation performed on that stream is physically reflected in the file.
To operate with streams we have to our disposal the standard iostream library
which provides us the following elements:
- Basic class templates
- The base of the iostream library is the hierarchy of class templates.
The class templates provide most of the functionality of the library in a way
that they can be used to operate with any type of elements.
This is a set of class templates have two template parameters: the char type
(charT) that determines the type of elements to be manipulated and the
traits, that provides additional characteristics specific
for that type of elements.
The class templates in this hierarchy have the same name as their char-type
instantiations but with basic_ as prefix. For example, the class template which
istream is instantiated from is called basic_istream, the one of fstream
is basic_fstream and so on. The only exception is ios_base.
- Class template instantiations
- The library incorporates two standard sets of instantiations of the iostream class
template hierarchy:
one, narrow-oriented, to manipulate char elements and another one,
wide-oriented, to manipulate wchar_t elements.
The narrow-oriented (char) instantiation is probably the better known part of
the iostream library. Classes like ios, istream and
ofstream are narrow-oriented. The diagram on top of this page shows the
names and relationships of narrow-oriented classes.
The classes of the wide-oriented (wchar_t) instatiation use the same names
as the narrow-oriented but prefixed with a w character, like wios,
wistream and wofstream.
- Standard objects
-
Within the iostream library, declared in the <iostream>
header file there are certain objects that are used to perform input and output
operations on the standard input and output devices.
They are divided in two sets: narrow-oriented objects, which are the popular cin,
cout, cerr and clog and
wide-oriented iostream objects, declared as wcin, wcout, wcerr
and wclog.
- Types
-
The iostream classes barely use fundamental types on their member's prototypes.
They generally use defined types that depend on the traits used in the
instantiation.
For the default char and wchar_t specializations, types
streampos,
streamoff and
streamsize
are used to represent positions, offsets and sizes, respectivelly.
- Manipulators
-
Manipulators are global functions designed to be used
in conjunction with insertion (<<) and extraction (>>)
operators of stream objects. They generally modify properties and formatting
information of streams.
endl, hex and scientific are examples of manipulators.
Organization:
The library and its hierarchy of classes is divided in different files:
-
<ios>,
<istream>,
<ostream>,
<streambuf> and
<iosfwd>
aren't usually included directly in most C++ programs. They describe the base classes
of the hierarchy and are automatically included by other header files of the library
that contain the derived classes.
-
<iostream>
declares the objects used to communicate through the standard input and output
(including cin and out).
-
<fstream>
defines the file stream classes (like template basic_ifstream or class
ofstream) as well as the internal buffer objects used (basic_filebuf).
These classes are used to manipulate files with streams.
-
<sstream>.
The classes defined in this file are used to manipulate STL string objects as if they
were streams.
-
<iomanip> declares some standard manipulators with
parameters to be used with extraction and insertion operators to modify internal flags and
formatting options.
Compatibility notes
1. The names, prototypes and examples included in this reference for the iostream classes
mostly describe and use the char instatiations of the class templates instead of the
templates themselves. Although these classes are only one of the possible instantiations
of the templates, this has been done thus in order to provide a better readability
and assuming it is as easy to obtain the names and prototypes of the basic template from
the char specialization as the opposite.
2. The classes described in this reference follow the ANSI C++ standard.
Many compilers released before the publication of this standard may have a
different implementation which is not considered in this reference.
The C++ FAQ includes an example
to check if your compiler may be ANSI compliant.
|
© The C++ Resources Network, 2001
|